Introduction to the C Language
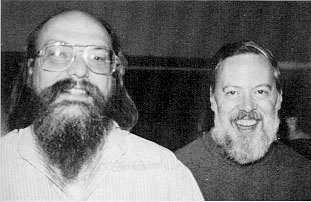
Right: Dennis Ritchie, developer of C
The C Programming language was developed by Dennis Ritchie in 1972 while he was working at AT&T (then known as Bell Telephone Laboratories). Ritchie based his new C language on a prior one called B, which was written by his colleague Ken Thompson. By 1973 the powerful Unix operating system was written entirely in C.
Today the C programming language is still quite popular. In fact, many modern programming languages, such as Java, C++, and even Google's “GO” programming language, were based on various features of the C language.
The C programming language is mainly known for its flexibility and power; plus C is modular based, using functions for routines, which helps programmers build reusable code. It's also known for its recursion abilities, low-level access, string manipulations, math functions, and its “structured programming” qualities that entirely remove GOTO statements, thus cutting down on programmers writing ugly “spaghetti code.” C is also known for having very few constraints, hence any type of program can be written with it, from word processing software to fancy graphics to entire operating systems. C is also a small language, meaning it doesn't have many keywords, yet it is still extremely powerful. (One drawback to C is that it doesn't have “garbage collection” which is a form of automatically reclaiming any unused memory.)
For all of these abundant qualities (and many more), C quickly became extremely popular beyond the confines of AT&T (Bell Telephone Laboratories). But with each new company or facility that used C, they made various changes to the overall language in numerous ways, which eventually created problems. Soon an “ANSI” committee was established (American National Standards Institute) in 1983 to develop a universal standard of the C language, which solved many portability issues. A classic book titled, “The C Programming Language” by Dennis Ritchie and Brian Kernighan was published in 1978, which is still a strong seller today.
To write a C program you will need only two tools: a text editor, and a compiler. A C program is called “source code” and can be written using any word processor that you have handy; e. g. Notepad in Windows, Vi in Unix, or another text editor. Simply type in your code and save it with a .c extension, then compile and link the code (linking is usually done automatically by the compiler and is a process of combining the source code with certain predefined functions, turning the .c program into an .obj file, and finally into an .exe file,) which makes the original .c program into a series of machine language instructions that can be used (executed) by the computer.
If you would like to learn the C language, or simply experiment with some simple functions and observe its capabilities, there are many free C compilers out there waiting to be downloaded. One such compiler is called “Pacific C,” which is free and easy to get up and running. Here is an example program that will run in Pacific C (it's easy to figure out its basic function). Type it up and run it with Pacific C or another compiler of your choice:
int radius, area;
main()
{
printf("Enter your radius (i.e. 10): ");
scanf("%d", &radius);
area = 3.141592653 * radius * radius;
printf("\n\nThe total area computed is: %d\n\n", area);
return(0);
}
So there you have a brief history of the C programming language and even an example program to get you started if you would like to see if programming is for you. Although programming can be quite tedious at times, there's a definite sense of satisfaction one feels after getting a complicated program to run correctly. Who knows, you may discover you enjoy programming immensely and would like to learn more so that eventually you can procure a high-paying job in the IT industry.